1. 打印命令行提示符
#include<stdlib.h> char* HostName() //获取主机名 { char* ret = getenv("HOSTNAME"); if(ret) return ret; else return (char*)"None"; } char* UserName() //获取用户名 { char* ret = getenv("USER"); if(ret) return ret; else return (char*)"None"; } char* CurrentWorkdir() //获取当前工作目录 { char* ret = getenv("PWD"); if(ret) return ret; else return (char*)"None"; } int main() { //打印命令行提示符 //读取环境变量的内容 / 调用函数(gethostname获取主机名、getcwd获取当前工作目录) printf("[%s@%s %s]#", UserName(), HostName(), CurrentWorkdir()); }
2. 获取用户输入的命令行字符串
1.可以使用scanf读取字符串吗?
答:不能,scanf用于从标准输入(键盘)读取格式化输入,读取字符串时,scanf会在遇到空白字符(空格、换行符\n、制表符\t等)时停止读取,并将读取的字符串存储到字符数组中。
1.概念:是shell内部直接提供并实现的命令,不要shell创建子进程来调用一个独立的外部程序(exe函数)来执行,而是由shell本身解释并执行。
2.可用性和依赖性:内建命令随shell的安装而自动提供,不需要额外的安装。
3.常见的内建命令:cd、echo、export、pwd、exit等。
4.2. 外部命令
4.3. cd
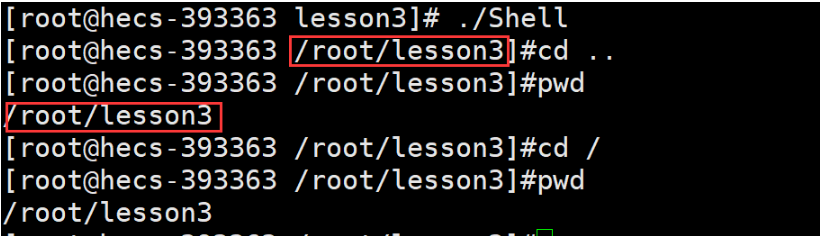
int BuiltinCmd() { int ret = 0; //用于判断是否为内建命令,不是,则为0、是,则为1 if(strcmp("cd", argv[0]) == 0) { ret = 1; char* target = argv[1]; //需要切换的路径 if(!target) target = getenv("HOME"); //cd,表示切换到家目录中 chdir(target); //改变当前工作目录,但不改变环境变量PWD的内容 char tmp[SIZE]; snprintf(tmp, SIZE, "PWD=%s", target); //多变一 putenv(tmp); //修改或者新增环境变量 } return ret; }
#include<stdio.h> #include<stdlib.h> #include<string.h> #include<unistd.h> #define SIZE 1024 char* argv[SIZE]; //相当于main函数的命令行参数表,末尾一定要以NULL结尾 int BuiltinCmd() { int ret = 0; //用于判断是否为内建命令,不是,则为0、是,则为1 if(strcmp("cd", argv[0]) == 0) { ret = 1; char* target = argv[1]; //需要切换的路径 if(!target) target = getenv("HOME"); //cd,表示切换到家目录中 chdir(target); //更改当前工作目录 char tmp[SIZE]; //存储获取到的当前工作目录的绝对路径 char pwd[SIZE]; //存储更改后的环境变量PWD的内容 getcwd(tmp, SIZE); //获取当前工作目录的绝对路径 snprintf(pwd, SIZE, "PWD=%s", tmp); //多变一,拼接 putenv(pwd); //修改环境变量PWD的内容 } return ret; } int main() { char CommandLine[SIZE]; while(1) { //1.打印命令行提示符+获取用户输入的命令行字符串 int n = Interative(CommandLine); if(n == 0) continue; //空串,用户继续输入 //2.分割命令行字符串 Split(CommandLine); //3.处理内建命令 n = BuiltinCmd(); if(n) continue; } return 0; }
4.5. export
int BuiltinCmd() { int ret = 0; if(strcmp("export", argv[0]) == 0) { ret = 1; if(argv[1]) { putenv(argv[1]); } } return ret; }
char env[SIZE]; //相当于环境变量表,存储新增的环境变量 int BuiltinCmd() { if(strcmp("export", argv[0]) == 0) int ret = 0; { ret = 1; if(argv[1]) { strcpy(env, argv[1]); //将新增的环境变量的内容,拷贝到环境变量表中 putenv(env); } } return ret; }
4.6. echo
char env[SIZE]; //相当于环境变量表,存储新增的环境变量 int BuiltinCmd() { if(strcmp("echo", argv[0]) == 0) { ret = 1; if(!argv[1]) //echo:打印一个空行 printf("\n"); else if(argv[1][0] != '$') printf("%s\n", argv[1]); //echo aaaa:打印aaaa else { if(argv[1][1] == '?') { printf("%d\n", exit_code); //echo $?:打印退出码 exit_code = 0; //便于在执行echo $?时,退出码=0 } else printf("%s\n", getenv(argv[1] + 1)); //echo $USER:打印环境变量的内容 } } return ret; }
5. 执行命令
5.1. 创建子进程进行程序替换
#include<stdlib.h> #include<string.h> #include<sys/types.h> #include<sys/wait.h> #define SIZE 1024 #define SP " " char* argv[SIZE]; int exit_code; //全局变量,存储子进程的退出码,便于echo $?直接输出退出码 void Execute() { pid_t id = fork(); if(id == 0) { execvp(argv[0], argv); //程序替换,执行命令 exit(1); //子进程退出 } int status = 0; pid_t rid = waitpid(id, &status, 0); //父进程等待,进行回收子进程的资源 if(rid > 0) { exit_code = WEXITSTATUS(status); //正常退出,获取子进程的退出码 } } int main() { char CommandLine[SIZE]; while(1) { //1.打印命令行提示符+获取用户输入的命令行字符串 int n = Interative(CommandLine); if(n == 0) continue; //空串,用户继续输入 //2.分割命令行字符串 Split(CommandLine); //4.执行命令(创建子进程,进行替换) Execute(); } return 0; }
6. 重定向
为什么要宏定义多个整数,定义全局变量redir_type?
重定向有三种类型:输出重定向>、追加重定向>>、输出重定向。
我们需要在命令行参数中,查找是否有重定向,如果有重定向,就需要获取重定向的类型,从而在根据重定向的类型执行对应的指令。
所以我们用宏定义多个整数,来表示重定向的类型; 定义一个全局变量redir_type,用来记录获取到的重定向的类型
#define NoneRedir -1 #define StdinRedir 0 #define StdoutRedir 1 #define AppenRedir 2 char* filename = NULL; int redir_type = -1;
3.执行流程:首先判断是否包含重定向(checkRedir);再把我们要执行的命令进行分分割(将重定向字符设置为’\0’,这样strtok只能切割要执行的命令);最后让子进程执行重定向操作。
#define IsSpace(buf, pos) do{while(isspace(buf[pos])) pos ++;}while(0)
//用宏定义多个整数,来表示重定向的类型 #define NoneRedir -1 #define StdinRedir 0 #define StdoutRedir 1 #define AppenRedir 2 char* filename = NULL; //记录文件名 int redir_type = -1; //记录重定向类型 //去除命令行参数中的空格(连续空格) #define IsSpace(buf, pos) do{while(isspace(buf[pos])) pos++;}while(0) //检查是否包含重定向符号 void CheckRedir(char* in) { filename = NULL; redir_type = -1; int pos = strlen(in) - 1; //从命令行参数字符串后面往前找 while(pos >= 0) { if(in[pos] == '>') { if(in[pos - 1] == '>') //追加重定向 { redir_type = 2; in[pos - 1] = '\0'; //便于将要执行的命令分割开 pos++; //当前pos位置不是空格,isspace函数为假,直接返回 IsSpace(in, pos); //跳过空格 filename = in + pos; //获取文件名 break; //记录完毕,就立即退出 } else //输出重定向 { redir_type = 1; in[pos] = '\0'; pos++; IsSpace(in, pos); filename = in + pos; break; } } else if(in[pos] == '<') //输入重定向 { redir_type = 0; in[pos] = '\0'; pos++; IsSpace(in, pos); filename = in + pos; break; } else { pos--; } } } void Split(char* in) { CheckRedir(in); //在分割之前,先判断是否包含重定向符号 int i = 0; argv[i++] = strtok(in, SP); //首次调用 while(argv[i++] = strtok(NULL, SP)); //后续调用 if(strcmp("ls", argv[0]) == 0) { argv[i-1] = (char*)"--color"; //加上后,ls显示内容,颜色为auto argv[i] = NULL; //argv以NULL结尾,便于子进程在进程程序替换时参数的传递 } } void Execute() { pid_t id = fork(); if(id == 0) { //让子进程执行重定向 int fd = -1; if(redir_type == StdinRedir) { fd = open(filename, O_RDONLY); dup2(fd, 0); //改变文件描述符的执行,从而实现输入、输出重定向 } else if(redir_type == StdoutRedir) { fd = open(filename, O_WRONLY|O_CREAT|O_TRUNC, 0666); dup2(fd, 1); } else if(redir_type == AppenRedir) { fd = open(filename, O_WRONLY|O_CREAT|O_APPEND , 0666); dup2(fd, 1); } execvp(argv[0], argv); //程序替换,执行命令 exit(1); //子进程退出 } int status = 0; pid_t rid = waitpid(id, &status, 0); //父进程等待,进行回收子进程的资源 if(rid > 0) { exit_code = WEXITSTATUS(status); //正常退出,获取子进程的退出码 } }
7. 总代码
#include<stdio.h> #include<stdlib.h> #include<string.h> #include<unistd.h> #include<sys/types.h> #include<sys/wait.h> #include<ctype.h> #include<sys/stat.h> #include<fcntl.h> #define SIZE 1024 #define SP " " char* argv[SIZE]; char env[SIZE]; //相当于环境变量表,存储新增的环境变量 int exit_code; //全局变量,存储子进程的退出码,便于echo $?直接输出退出码 //以下都和重定向有关 //用宏定义多个整数,来表示重定向的类型 #define NoneRedir -1 #define StdinRedir 0 #define StdoutRedir 1 #define AppenRedir 2 char* filename = NULL; //记录文件名 int redir_type = -1; //记录重定向类型 //去除命令行参数中的空格(连续空格) #define IsSpace(buf, pos) do{while(isspace(buf[pos])) pos++;}while(0) char* HostName() //获取主机名 { char* ret = getenv("HOSTNAME"); if(ret) return ret; else return (char*)"None"; } char* UserName() //获取用户名 { char* ret = getenv("USER"); if(ret) return ret; else return (char*)"None"; } char* CurrentWorkdir() //获取当前工作目录 { char* ret = getenv("PWD"); if(ret) return ret; else return (char*)"None"; } int Interative(char* in) { printf("[%s@%s %s]#", UserName(), HostName(), CurrentWorkdir()); fgets(in, SIZE, stdin); in[strlen(in) - 1] = '\0'; //移除换行字符\n return strlen(in) - 1; //处理空串""情况 } //检查是否包含重定向符号 void CheckRedir(char* in) { filename = NULL; redir_type = -1; int pos = strlen(in) - 1; //从命令行参数字符串后面往前找 while(pos >= 0) { if(in[pos] == '>') { if(in[pos - 1] == '>') //追加重定向 { redir_type = 2; in[pos - 1] = '\0'; //便于将要执行的命令分割开 pos++; //当前pos位置不是空格,isspace函数为假,直接返回 IsSpace(in, pos); //跳过空格 filename = in + pos; //获取文件名 break; //记录完毕,就立即退出 } else //输出重定向 { redir_type = 1; in[pos] = '\0'; pos++; IsSpace(in, pos); filename = in + pos; break; } } else if(in[pos] == '<') //输入重定向 { redir_type = 0; in[pos] = '\0'; pos++; IsSpace(in, pos); filename = in + pos; break; } else { pos--; } } } void Split(char* in) { CheckRedir(in); //在分割之前,先判断是否包含重定向符号 int i = 0; argv[i++] = strtok(in, SP); //首次调用 while(argv[i++] = strtok(NULL, SP)); //后续调用 if(strcmp("ls", argv[0]) == 0) { argv[i-1] = (char*)"--color"; //加上后,ls显示内容,颜色为auto argv[i] = NULL; //argv以NULL结尾,便于子进程在进程程序替换时参数的传递 } } int BuiltinCmd() { if(strcmp("echo", argv[0]) == 0) { ret = 1; if(!argv[1]) //echo:打印一个空行 printf("\n"); else if(argv[1][0] != '$') printf("%s\n", argv[1]); //echo aaaa:打印aaaa else { if(argv[1][1] == '?') { printf("%d\n", exit_code); //echo $?:打印退出码 exit_code = 0; //便于在执行echo $?时,退出码=0 } else printf("%s\n", getenv(argv[1] + 1)); //echo $USER:打印环境变量的内容 } } else if(strcmp("cd", argv[0]) == 0) { ret = 1; char* target = argv[1]; //需要切换的路径 if(!target) target = getenv("HOME"); //cd,表示切换到家目录中 chdir(target); //更改当前工作目录 char tmp[SIZE]; //存储获取到的当前工作目录的绝对路径 char pwd[SIZE]; //存储更改后的环境变量PWD的内容 getcwd(tmp, SIZE); //获取当前工作目录的绝对路径 snprintf(pwd, SIZE, "PWD=%s", tmp); //多变一,拼接 putenv(pwd); //修改环境变量PWD的内容 } else if(strcmp("export", argv[0]) == 0) int ret = 0; { ret = 1; if(argv[1]) { strcpy(env, argv[1]); //将新增的环境变量的内容,拷贝到环境变量表中 putenv(env); } } return ret; } void Execute() { pid_t id = fork(); if(id == 0) { //让子进程执行重定向 int fd = -1; if(redir_type == StdinRedir) { fd = open(filename, O_RDONLY); dup2(fd, 0); //改变文件描述符的执行,从而实现输入、输出重定向 } else if(redir_type == StdoutRedir) { fd = open(filename, O_WRONLY|O_CREAT|O_TRUNC, 0666); dup2(fd, 1); } else if(redir_type == AppenRedir) { fd = open(filename, O_WRONLY|O_CREAT|O_APPEND , 0666); dup2(fd, 1); } execvp(argv[0], argv); //程序替换,执行命令 exit(1); //子进程退出 } int status = 0; pid_t rid = waitpid(id, &status, 0); //父进程等待,进行回收子进程的资源 if(rid > 0) { exit_code = WEXITSTATUS(status); //正常退出,获取子进程的退出码 } } int main() { char CommandLine[SIZE]; while(1) { //1.打印命令行提示符+获取用户输入的命令行字符串 int n = Interative(CommandLine); if(n == 0) continue; //空串,用户继续输入 //2.分割命令行字符串 Split(CommandLine); //3.处理内建命令 n = BuiltinCmd(); if(n) continue; //4.执行命令(创建子进程,进行替换 Execute(); } return 0; }
本文链接:https://blog.runxinyun.com/post/100.html 转载需授权!
留言0